图书介绍
Thinking in C++ Volume 2: Practical Programming = C++编程思想 第2卷 实用编程技术 (英文版)2025|PDF|Epub|mobi|kindle电子书版本百度云盘下载
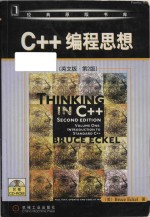
- Bruce Eckel ; Chuck Allison 著
- 出版社: China Machine Press
- ISBN:7111121880
- 出版时间:2002
- 标注页数:802页
- 文件大小:81MB
- 文件页数:823页
- 主题词:C语言-程序设计-英文
PDF下载
下载说明
Thinking in C++ Volume 2: Practical Programming = C++编程思想 第2卷 实用编程技术 (英文版)PDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
Preface1
What’s new in the second edition2
What’s in Volume 2 of this book3
How to get Volume 23
Prerequisites3
Learning C++4
Goals5
Chapters7
Exercises12
Exercise solutions12
Source code12
Language standards14
Language support15
The book’s CD ROM15
CD ROMs,seminars,and consulting16
Errors16
About the cover17
Book design and production18
Acknowledgements19
1:Introduction to Objects21
The progress of abstraction22
An object has an interface25
The hidden implementation28
Reusing the implementation29
Inheritance:reusing the interface31
Is-a vs.is-like-a relationships35
Interchangeable objects with polymorphism37
Creating and destroying objects41
Exception handling:dealing with errors43
Analysis and design44
Phase 0:Make a plan47
Phase 1:What a we making?48
Phase 2:How will we build it?52
Phase 3:Build the core56
Phase 4:Iterate the use cases57
Phase 5:Evolution58
Plans pay off60
Extreme programming61
Write tests first61
Pair programming63
Why C++ succeeds64
A better C65
You’re already on the learning curve65
Efficiency66
Systems are easier to express and understand66
Maximal leverage with libraries67
Source-code reuse with templates67
Error handling67
Programming in the large68
Strategies for transition68
Guidelines69
Management obstacles71
Summary73
2:Making & Using Objects75
The process of language translation76
Interpreters77
Compilers77
The compilation process79
Tools for separate compilation80
Declarations vs.definitions81
Linking87
Using libraries88
Your first C++ program90
Using the lostreams class90
Namespaces91
Fundamentals of program structure93
“Hello,world!”94
Running the compiler95
More about iostreams96
Character array concatenation96
Reading input97
Calling other programs98
Introducing strings98
Reading and writing files100
Introducing vector102
Summary108
Exercises109
3:The C in C++111
Creating functions112
Function return values115
Using the C function library116
Creating your own braries with the librarian117
Controlling execution117
True and false117
if-else118
while119
do-while120
for121
The break and continue keywords122
switch123
Using and misusing goto125
Recursion126
Introduction to operators127
Precedence127
Auto increment and decrement128
Introduction to data types129
Basic built-in types129
bool,true,& false131
Specifiers132
Introduction to pointers133
Modifying the outside object137
Introduction to C++ references140
Pointers and references as modifiers141
Scoping143
Defining variables on the fly145
Specifying storage allocation147
Global variables147
Local variables149
static149
extem151
Constants153
volatile155
Operators and their use156
Assignment156
Mathematical operators156
Relational operators158
Logical operators158
Bitwise operators159
Shift operators160
Unary operators163
The ternary operator164
The comma operator165
Common pitfalls when using operators166
Casting operators166
C++ explicit casts167
sizeof - an operator by itself172
The asm keyword173
Explicit operators173
Composite type creation174
Aliasing names with typedef174
Combining variables with struct175
Clarifying programs with enum179
Saving memory with union181
Arrays182
Debugging hints193
Debugging flags194
Turning variables and expressions into strings196
The C assert( ) macro197
Function addresses198
Defining a function pointer198
Complicated declarations & definitions199
Using a function pointer200
Arrays of pointers to functions201
Make:managing separate compilation202
Make activities204
Makefiles in this book207
An example makefile208
Summary210
Exercises210
4:Data Abstraction217
A tiny C-like library219
Dynamic storage allocation223
Bad guesses227
What’s wrong?229
The basic object230
What’s an object?238
Abstract data typing239
Object details240
Header file etiquette241
Importance of header files242
The multiple-declaration problem244
The preprocessor directives #define,#ifdef,and #endif245
A standard for header files246
Namespaces in headers247
Using headers in projects248
Nested structures248
Global scope resolution253
Summary253
Exercises254
5:Hiding the Implementation259
Setting limits260
C++ access control261
protected263
Friends263
Nested friends266
Is it pure?269
Object layout269
The class270
Modifying Stash to use access control273
Modifying Stack to use access control274
Handle classes275
Hiding the implementation276
Reducing recompilation276
Summary279
Exercises280
6:Initialization &Cleanup283
Guaranteed initialization with the constructor285
Guaranteed cleanup with the destructor287
Elimination of the definition block289
for loops291
Storage allocation292
Stash with constructors and destructors294
Stack with constructors & destructors298
Aggregate initialization301
Default constructors304
Summary305
Exercises306
7:Function Overloading & Default Arguments309
More name decoration311
Overloading on return values312
Type-safe linkage313
Overloading example314
unions318
Default arguments321
Placeholder arguments323
Choosing overloading vs.default arguments324
Summary329
Exercises330
8:Constants333
Value substitution334
const in header files335
Safety consts336
Aggregates337
Differences with C338
Pointers340
Pointer to const340
const pointer341
Assignment and pe checking343
Function arguments & return values344
Passing by const value344
Returning by const value345
passing and returning addresses349
Classes352
const in classes353
Compile-time constants in classes356
const objects &member functions359
volatile365
Summary367
Exercises367
9:Inline Functions371
Preprocessor pitfalls372
Macros and access376
Inline functions377
Inlines inside classes378
Access functions379
Stash & Stack with inlines385
Inlines &the compiler390
Limitations390
Forward references391
Hidden activities in constructors &destructors392
Reducing clutter393
More preprocessor features395
Token pasting396
Improved error checking396
Summary400
Exercises400
10:Name Control405
Static elements from C406
static variables inside functions406
Controlling linkage412
Other storage class specifiers414
Namespaces414
Creating a namespace415
Using a namespace417
The use of namespaces422
Static members in C++423
Defining storage for static data members424
Nested and local classes428
static member functions429
Static initialization dependency432
What to do434
Alternate linkage specifications442
Summary443
Exercises443
11:References & the Copy-Constructor449
Pointers in C++450
References in C++451
References in functions452
Argument-passing guidelines455
The copy-constructor455
Passing & returning by value455
Copy-construction462
Default copy-constructor468
Altematives to copy-construction471
Pointers to members473
Functions475
Summary478
Exercises479
12:Operator Overloading485
Warning &reassurance486
Syntax487
Overloadable operators488
Unary operators489
Binary operators493
Arguments &return values505
Unusual operators508
Operators you can’t overload517
Non-member operators518
Basic guidelines520
Overloading assignment521
Behavior of operator=522
Automatic type conversion533
Constructor conversion534
Operator conversion535
Type conversion example538
Pitfalls in automatic type conversion539
Summary542
Exercises542
13:Dynamic Object Creation547
Object creation549
C’s approach to the heap550
operator new552
operator delete553
A simple example553
Memory manager overhead554
Early examples redesigned555
delete void* is probably a bug555
Cleanup responsibility with pointers557
Stash for pointers558
new & delete for arrays563
Making a pointer more like an array564
Running out of storage565
Overloading new & delete566
Overloading global new & delete568
Overloading new & delete for a class570
Overloading new & delete for arrays573
Constructor calls576
placement new & delete577
Summary580
Exercises580
14:Inheritance & Composition583
Composition syntax584
Inheritance syntax586
The constructor initializer list588
Member object initialization589
Built-in types in the initializer list589
Combining composition & inheritance591
Order of constructor &destructor calls592
Name hiding595
Functions that don’t automatically inherit600
Inheritance and static member functions604
Choosing composition vs.inheritance604
Subtyping606
private inheritance609
protected610
protected inheritance611
Operator overloading & inheritance612
Multiple inheritance613
Incremental development614
Upcasting615
Why “upcasting?”617
Upcasting and the copy-constructor617
Composition vs.inheritance (revisited)620
Pointer & reference upcasting622
A crisis622
Summary623
Exercises623
15:Polymorphism &Virtual Functions627
Evolution of C++programmers628
Upcasting629
The problem631
Function call binding631
virtual functions632
Extensibility633
How C++ implements late binding636
Storing type information637
Picturing virtual functions639
Under the hood642
Installing the vpointer643
Objects are different644
Why virtual functions?645
Abstract base classes and pure virtual functions646
Pure virtual definitions651
Inheritance and the VTABLE652
Object slicing655
Overloading &overriding658
Variant return type660
virtual functions &constructors662
Order of constructor calls663
Behavior of virtual functions inside constructors664
Destructors and virtual destructors665
Pure virtual destructors668
Virtuals in destructors670
Creating an object-based hierarchy671
Operator overloading675
Downcasting678
Summary681
Exercises682
16:Introduction to Templates689
Containers690
The need for containers692
Overview of templates693
The template solution696
Template syntax697
Non-inline function definitions699
IntStack as a template701
Constants in templates703
Stack and Stash as templates705
Templatized pointer Stash707
Turning ownership on and off713
Holding objects by value716
Introducing iterators719
Stack with iterators728
PStash with iterators732
Why iterators?738
Function templates742
Summary743
Exercises744
A:Coding Style747
B:Programming Guidelines759
C:Recommended Reading775
C776
General C++776
My own list of tooks777
Depth &dark corners778
Analysis & design779
Index783
热门推荐
- 687747.html
- 832652.html
- 2465307.html
- 1092927.html
- 2231295.html
- 290460.html
- 814117.html
- 1149342.html
- 1203708.html
- 1361575.html
- http://www.ickdjs.cc/book_1036418.html
- http://www.ickdjs.cc/book_679181.html
- http://www.ickdjs.cc/book_3848944.html
- http://www.ickdjs.cc/book_504774.html
- http://www.ickdjs.cc/book_2060369.html
- http://www.ickdjs.cc/book_437641.html
- http://www.ickdjs.cc/book_3541954.html
- http://www.ickdjs.cc/book_2009149.html
- http://www.ickdjs.cc/book_2176258.html
- http://www.ickdjs.cc/book_2352189.html