图书介绍
现代C++探秘 编码、工程与科研必修 基于C++14 英文版2025|PDF|Epub|mobi|kindle电子书版本百度云盘下载
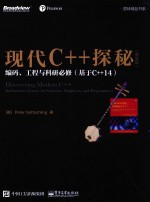
- (德)Peter Gottschling著 著
- 出版社: 北京:电子工业出版社
- ISBN:9787121308543
- 出版时间:2017
- 标注页数:444页
- 文件大小:68MB
- 文件页数:465页
- 主题词:C语言-程序设计-英文
PDF下载
下载说明
现代C++探秘 编码、工程与科研必修 基于C++14 英文版PDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
Chapter 1 C++ Basics1
1.1 Our First Program1
1.2 Variables3
1.2.1 Constants5
1.2.2 Literals6
1.2.3 Non-narrowing Initialization7
1.2.4 Scopes8
1.3 Operators10
1.3.1 Arithmetic Operators11
1.3.2 Boolean Operators14
1.3.3 Bitwise Operators15
1.3.4 Assignment15
1.3.5 Program Flow16
1.3.6 Memory Handling17
1.3.7 Access Operators17
1.3.8 Type Handling17
1.3.9 Error Handling18
1.3.10 Overloading18
1.3.11 Operator Precedence18
1.3.12 Avoid Side Effects !18
1.4 Expressions and Statements21
1.4.1 Expressions21
1.4.2 Statements21
1.4.3 Branching22
1.4.4 Loops24
1.4.5 goto27
1.5 Functions28
1.5.1 Arguments28
1.5.2 Returning Results30
1.5.3 Inlining31
1.5.4 Overloading31
1.5.5 main Function33
1.6 Error Handling34
1.6.1 Assertions34
1.6.2 Exceptions35
1.6.3 Static Assertions40
1.7 I/O40
1.7.1 Standard Output40
1.7.2 Standard Input41
1.7.3 Input/Output with Files41
1.7.4 Generic Stream Concept42
1.7.5 Formatting43
1.7.6 Dealing with I/O Errors44
1.8 Arrays,Pointers,and References47
1.8.1 Arrays47
1.8.2 Pointers49
1.8.3 Smart Pointers51
1.8.4 References55
1.8.5 Comparison between Pointers and References55
1.8.6 Do Not Refer to Outdated Data !55
1.8.7 Containers for Arrays56
1.9 Structuring Software Projects58
1.9.1 Comments59
1.9.2 Preprocessor Directives60
1.10 Exercises63
1.10.1 Age63
1.10.2 Arrays and Pointers64
1.10.3 Read the Header of a Matrix Market File64
Chapter 2 Classes65
2.1 Program for Universal Meaning Not for Technical Details65
2.2 Members67
2.2.1 Member Variables67
2.2.2 Accessibility68
2.2.3 Access Operators70
2.2.4 The Static Declarator for Classes70
2.2.5 Member Functions71
2.3 Setting Values:Constructors and Assignments72
2.3.1 Constructors72
2.3.2 Assignment81
2.3.3 Initializer Lists82
2.3.4 Uniform Initialization83
2.3.5 Move Semantics85
2.4 Destructors89
2.4.1 Implementation Rules89
2.4.2 Dealing with Resources Properly90
2.5 Method Generation Résumé95
2.6 Accessing Member Variables96
2.6.1 Access Functions96
2.6.2 Subscript Operator97
2.6.3 Constant Member Functions98
2.6.4 Reference-Qualified Members99
2.7 Operator Overloading Design100
2.7.1 Be Consistent !101
2.7.2 Respect the Priority101
2.7.3 Member or Free Function102
2.8 Exercises104
2.8.1 Polynomial104
2.8.2 Move Assignment104
2.8.3 Initializer List105
2.8.4 Resource Rescue105
Chapter 3 Generic Programming107
3.1 Function Templates107
3.1.1 Instantiation108
3.1.2 Parameter Type Deduction109
3.1.3 Dealing with Errors in Templates113
3.1.4 Mixing Types113
3.1.5 Uniform Initialization115
3.1.6 Automatic return Type115
3.2 Namespaces and Function Lookup115
3.2.1 Namespaces115
3.2.2 Argument-Dependent Lookup118
3.2.3 Namespace Qualification or ADL122
3.3 Class Templates123
3.3.1 A Container Example124
3.3.2 Designing Uniform Class and Function Interfaces125
3.4 Type Deduction and Definition131
3.4.1 Automatic Variable Type131
3.4.2 Type of an Expression132
3.4.3 decltype(auto)133
3.4.4 Defining Types134
3.5 A Bit of Theory on Templates:Concepts136
3.6 Template Specialization136
3.6.1 Specializing a Class for One Type137
3.6.2 Specializing and Overloading Functions139
3.6.3 Partial Specialization141
3.6.4 Partially Specializing Functions142
3.7 Non-Type Parameters for Templates144
3.8 Functors146
3.8.1 Function-like Parameters148
3.8.2 Composing Functors149
3.8.3 Recursion150
3.8.4 Generic Reduction153
3.9 Lambda154
3.9.1 Capture155
3.9.2 Capture by Value156
3.9.3 Capture by Reference157
3.9.4 Generalized Capture158
3.9.5 Generic Lambdas159
3.10 Variadic Templates159
3.11 Exercises161
3.11.1 String Representation161
3.11.2 String Representation of Tuples161
3.11.3 Generic Stack161
3.11.4 Iterator of a Vector162
3.11.5 Odd Iterator162
3.11.6 Odd Range162
3.11.7 Stack of bool162
3.11.8 Stack with Custom Size163
3.11.9 Deducing Non-type Template Arguments163
3.11.10 Trapezoid Rule163
3.11.11 Functor164
3.11.12 Lambda164
3.11.13 Implement make unique164
Chapter 4 Libraries165
4.1 Standard Template Library165
4.1.1 Introductory Example166
4.1.2 Iterators166
4.1.3 Containers171
4.1.4 Algorithms179
4.1.5 Beyond Iterators185
4.2 Numerics186
4.2.1 Complex Numbers186
4.2.2 Random Number Generators189
4.3 Meta-programming198
4.3.1 Limits198
4.3.2 Type Traits200
4.4 Utilities202
4.4.1 Tuple202
4.4.2 function205
4.4.3 Reference Wrapper207
4.5 The Time Is Now209
4.6 Concurrency211
4.7 Scientific Libraries Beyond the Standard213
4.7.1 Other Arithmetics214
4.7.2 Interval Arithmetic214
4.7.3 Linear Algebra214
4.7.4 Ordinary Differential Equations215
4.7.5 Partial Differential Equations215
4.7.6 Graph Algorithms215
4.8 Exercises215
4.8.1 Sorting by Magnitude215
4.8.2 STL Container216
4.8.3 Complex Numbers216
Chapter 5 Meta-Programming219
5.1 Let the Compiler Compute219
5.1.1 Compile-Time Functions219
5.1.2 Extended Compile-Time Functions221
5.1.3 Primeness223
5.1.4 How Constant Are Our Constants?225
5.2 Providing and Using Type Information226
5.2.1 Type Traits226
5.2.2 Conditional Exception Handling229
5.2.3 A const-Clean View Example230
5.2.4 Standard Type Traits237
5.2.5 Domain-Specific Type Properties237
5.2.6 enable-if239
5.2.7 Variadic Templates Revised242
5.3 Expression Templates245
5.3.1 Simple Operator Implementation245
5.3.2 An Expression Template Class248
5.3.3 Generic Expression Templates251
5.4 Meta-Tuning:Write Your Own Compiler Optimization253
5.4.1 Classical Fixed-Size Unrolling254
5.4.2 Nested Unrolling257
5.4.3 Dynamic Unrolling-Warm-up263
5.4.4 Unrolling Vector Expressions265
5.4.5 Tuning an Expression Template266
5.4.6 Tuning Reduction Operations269
5.4.7 Tuning Nested Loops276
5.4.8 Tuning Résumé282
5.5 Exercises283
5.5.1 Type Traits283
5.5.2 Fibonacci Sequence283
5.5.3 Meta-Program for Greatest Common Divisor283
5.5.4 Vector Expression Template284
5.5.5 Meta-List285
Chapter 6 Object-Oriented Programming287
6.1 Basic Principles287
6.1.1 Base and Derived Classes288
6.1.2 Inheriting Constructors291
6.1.3 Virtual Functions and Polymorphic Classes292
6.1.4 Functors via Inheritance297
6.2 Removing Redundancy298
6.3 Multiple Inheritance299
6.3.1 Multiple Parents300
6.3.2 Common Grandparents301
6.4 Dynamic Selection by Sub-typing306
6.5 Conversion308
6.5.1 Casting between Base and Derived Classes309
6.5.2 const-Cast313
6.5.3 Reinterpretation Cast313
6.5.4 Function-Style Conversion314
6.5.5 Implicit Conversions315
6.6 CRTP316
6.6.1 A Simple Example316
6.6.2 A Reusable Access Operator318
6.7 Exercises320
6.7.1 Non-redundant Diamond Shape320
6.7.2 Inheritance Vector Class320
6.7.3 Clone Function320
Chapter 7 Scientific Projects321
7.1 Implementation of ODE Solvers321
7.1.1 Ordinary Differential Equations321
7.1.2 Runge-Kutta Algorithms323
7.1.3 Generic Implementation325
7.1.4 Outlook331
7.2 Creating Projects332
7.2.1 Build Process333
7.2.2 Build Tools337
7.2.3 Separate Compilation340
7.3 Some Final Words345
Appendix A Clumsy Stuff347
A.1 More Good and Bad Scientific Software347
A.2 Basics in Detail353
A.2.1 More about Qualifying Literals353
A.2.2 static Variables355
A.2.3 More about if355
A.2.4 Duff's Device357
A.2.5 More about main357
A.2.6 Assertion or Exception?358
A.2.7 Binary I/O359
A.2.8 C-Style I/O360
A.2.9 Garbarge Collection360
A.2.10 Trouble with Macros361
A.3 Real-World Example:Matrix Inversion362
A.4 Class Details371
A.4.1 Pointer to Member371
A.4.2 More Initialization Examples372
A.4.3 Accessing Multi-dimensional Arrays373
A.5 Method Generation375
A.5.1 Controlling the Generation377
A.5.2 Generation Rules378
A.5.3 Pitfalls and Design Guides383
A.6 Template Details386
A.6.1 Uniform Initialization386
A.6.2 Which Function Is Called?386
A.6.3 Specializing for Specific Hardware389
A.6.4 Variadic Binary I/O390
A.7 Using std::vector in C++03391
A.8 Dynamic Selection in Old Style392
A.9 Meta-Programming Details392
A.9.1 First Meta-Program in History392
A.9.2 Meta-Functions394
A.9.3 Backward-Compatible Static Assertion396
A.9.4 Anonymous Type Parameters397
A.9.5 Benchmark Sources of Dynamic Unrolling400
A.9.6 Benchmark for Matrix Product401
Appendix B Programming Tools403
B.1 gcc403
B.2 Debugging404
B.2.1 Text-Based Debugger404
B.2.2 Debugging with Graphical Interface:DDD406
B.3 Memory Analysis408
B.4 gnuplot409
B.5 Unix,Linux,and Mac OS411
Appendix C Language Definitions413
C.1 Value Categories413
C.2 Operator Overview413
C.3 Conversion Rules416
C.3.1 Promotion416
C.3.2 Other Conversions416
C.3.3 Usual Arithmetic Conversions417
C.3.4 Narrowing418
Bibliography419
Index423
热门推荐
- 2237544.html
- 3328226.html
- 1000563.html
- 1813384.html
- 1902734.html
- 1443326.html
- 3765567.html
- 3797793.html
- 1779996.html
- 2526397.html
- http://www.ickdjs.cc/book_2176301.html
- http://www.ickdjs.cc/book_3024642.html
- http://www.ickdjs.cc/book_1107613.html
- http://www.ickdjs.cc/book_1446578.html
- http://www.ickdjs.cc/book_3319636.html
- http://www.ickdjs.cc/book_3403290.html
- http://www.ickdjs.cc/book_2569916.html
- http://www.ickdjs.cc/book_3618516.html
- http://www.ickdjs.cc/book_2938440.html
- http://www.ickdjs.cc/book_271671.html